Difference between modes a, a+, w, w+, and r+ in built-in open function?
Question:
In the python built-in open function, what is the exact difference between the modes w
, a
, w+
, a+
, and r+
?
In particular, the documentation implies that all of these will allow writing to the file, and says that it opens the files for “appending”, “writing”, and “updating” specifically, but does not define what these terms mean.
Answers:
The opening modes are exactly the same as those for the C standard library function fopen()
.
The BSD fopen
manpage defines them as follows:
The argument mode points to a string beginning with one of the following
sequences (Additional characters may follow these sequences.):
``r'' Open text file for reading. The stream is positioned at the
beginning of the file.
``r+'' Open for reading and writing. The stream is positioned at the
beginning of the file.
``w'' Truncate file to zero length or create text file for writing.
The stream is positioned at the beginning of the file.
``w+'' Open for reading and writing. The file is created if it does not
exist, otherwise it is truncated. The stream is positioned at
the beginning of the file.
``a'' Open for writing. The file is created if it does not exist. The
stream is positioned at the end of the file. Subsequent writes
to the file will always end up at the then current end of file,
irrespective of any intervening fseek(3) or similar.
``a+'' Open for reading and writing. The file is created if it does not
exist. The stream is positioned at the end of the file. Subse-
quent writes to the file will always end up at the then current
end of file, irrespective of any intervening fseek(3) or similar.
The options are the same as for the fopen function in the C standard library:
w
truncates the file, overwriting whatever was already there
a
appends to the file, adding onto whatever was already there
w+
opens for reading and writing, truncating the file but also allowing you to read back what’s been written to the file
a+
opens for appending and reading, allowing you both to append to the file and also read its contents
I hit upon this trying to figure out why you would use mode ‘w+’ versus ‘w’. In the end, I just did some testing. I don’t see much purpose for mode ‘w+’, as in both cases, the file is truncated to begin with. However, with the ‘w+’, you could read after writing by seeking back. If you tried any reading with ‘w’, it would raise an IOError. Reading without using seek with mode ‘w+’ isn’t going to yield anything, since the file pointer will be after where you have written.
I noticed that every now and then I need to Google fopen all over again, just to build a mental image of what the primary differences between the modes are. So, I thought a diagram will be faster to read next time. Maybe someone else will find that helpful too.
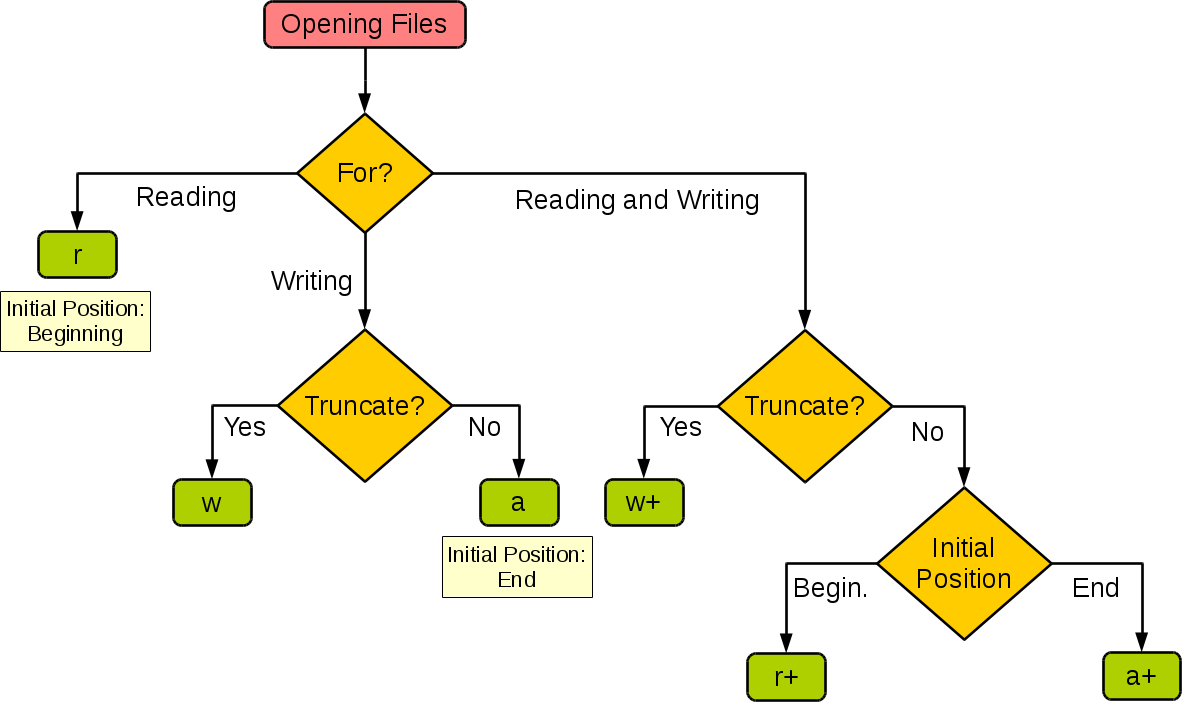
Same info, just in table form
| r r+ w w+ a a+
------------------|--------------------------
read | + + + +
write | + + + + +
write after seek | + + +
create | + + + +
truncate | + +
position at start | + + + +
position at end | + +
where meanings are:
(just to avoid any misinterpretation)
-
read – reading from file is allowed
-
write – writing to file is allowed
-
create – file is created if it does not exist yet
-
truncate – during opening of the file it is made empty (all content of the file is erased)
-
position at start – after file is opened, initial position is set to the start of the file
-
position at end – after file is opened, initial position is set to the end of the file
Note: a
and a+
always append to the end of file – ignores any seek
movements.
BTW. interesting behavior at least on my win7 / python2.7, for new file opened in a+
mode:
write('aa'); seek(0, 0); read(1); write('b')
– second write
is ignored
write('aa'); seek(0, 0); read(2); write('b')
– second write
raises IOError
I think this is important to consider for cross-platform execution, i.e. as a CYA. 🙂
On Windows, ‘b’ appended to the mode opens the file in binary mode, so there are also modes like ‘rb’, ‘wb’, and ‘r+b’. Python on Windows makes a distinction between text and binary files; the end-of-line characters in text files are automatically altered slightly when data is read or written. This behind-the-scenes modification to file data is fine for ASCII text files, but it’ll corrupt binary data like that in JPEG or EXE files. Be very careful to use binary mode when reading and writing such files. On Unix, it doesn’t hurt to append a ‘b’ to the mode, so you can use it platform-independently for all binary files.
This is directly quoted from Python Software Foundation 2.7.x.
I find it important to note that python 3 defines the opening modes differently to the answers here that were correct for Python 2.
The Python 3 opening modes are:
'r' open for reading (default)
'w' open for writing, truncating the file first
'x' open for exclusive creation, failing if the file already exists
'a' open for writing, appending to the end of the file if it exists
----
'b' binary mode
't' text mode (default)
'+' open a disk file for updating (reading and writing)
'U' universal newlines mode (for backwards compatibility; should not be used in new code)
The modes r
, w
, x
, a
are combined with the mode modifiers b
or t
. +
is optionally added, U
should be avoided.
As I found out the hard way, it is a good idea to always specify t
when opening a file in text mode since r
is an alias for rt
in the standard open()
function but an alias for rb
in the open()
functions of all compression modules (when e.g. reading a *.bz2
file).
Thus the modes for opening a file should be:
rt
/ wt
/ xt
/ at
for reading / writing / creating / appending to a file in text mode and
rb
/ wb
/ xb
/ ab
for reading / writing / creating / appending to a file in binary mode.
Use +
as before.
r
r+
x
x+
w
w+
a
a+
readable
x
x
x
x
x
writeable
x
x
x
x
x
x
x
default position: start
x
x
x
x
x
x
default position: end
x
x
must exist
x
x
mustn’t exist
x
x
truncate (clear file) on load
x
x
Always write to EOF
x
x
Mode
t (default)
b
str
(io.TextIOBase
)
x
bytes
(io.BufferedIOBase
)
x
If no mode is selected; text mode (t
) is used. As such r
is the same as rt
.
The particular doubt that many people get is ‘What is the difference between r+ and w+ modes?
The r+ helps you read and write data onto an already existing file without truncating (Error if there is no such file).
The w+ mode on the other hand also allows reading and writing but it truncates the file (if no such file exists – a new file is created). If you are wondering how it is possible to read from a truncated file, the reading methods can be used to read the newly written file (or the empty file).
Cheers!
In the python built-in open function, what is the exact difference between the modes w
, a
, w+
, a+
, and r+
?
In particular, the documentation implies that all of these will allow writing to the file, and says that it opens the files for “appending”, “writing”, and “updating” specifically, but does not define what these terms mean.
The opening modes are exactly the same as those for the C standard library function fopen()
.
The BSD fopen
manpage defines them as follows:
The argument mode points to a string beginning with one of the following
sequences (Additional characters may follow these sequences.):
``r'' Open text file for reading. The stream is positioned at the
beginning of the file.
``r+'' Open for reading and writing. The stream is positioned at the
beginning of the file.
``w'' Truncate file to zero length or create text file for writing.
The stream is positioned at the beginning of the file.
``w+'' Open for reading and writing. The file is created if it does not
exist, otherwise it is truncated. The stream is positioned at
the beginning of the file.
``a'' Open for writing. The file is created if it does not exist. The
stream is positioned at the end of the file. Subsequent writes
to the file will always end up at the then current end of file,
irrespective of any intervening fseek(3) or similar.
``a+'' Open for reading and writing. The file is created if it does not
exist. The stream is positioned at the end of the file. Subse-
quent writes to the file will always end up at the then current
end of file, irrespective of any intervening fseek(3) or similar.
The options are the same as for the fopen function in the C standard library:
w
truncates the file, overwriting whatever was already there
a
appends to the file, adding onto whatever was already there
w+
opens for reading and writing, truncating the file but also allowing you to read back what’s been written to the file
a+
opens for appending and reading, allowing you both to append to the file and also read its contents
I hit upon this trying to figure out why you would use mode ‘w+’ versus ‘w’. In the end, I just did some testing. I don’t see much purpose for mode ‘w+’, as in both cases, the file is truncated to begin with. However, with the ‘w+’, you could read after writing by seeking back. If you tried any reading with ‘w’, it would raise an IOError. Reading without using seek with mode ‘w+’ isn’t going to yield anything, since the file pointer will be after where you have written.
I noticed that every now and then I need to Google fopen all over again, just to build a mental image of what the primary differences between the modes are. So, I thought a diagram will be faster to read next time. Maybe someone else will find that helpful too.
Same info, just in table form
| r r+ w w+ a a+
------------------|--------------------------
read | + + + +
write | + + + + +
write after seek | + + +
create | + + + +
truncate | + +
position at start | + + + +
position at end | + +
where meanings are:
(just to avoid any misinterpretation)
-
read – reading from file is allowed
-
write – writing to file is allowed
-
create – file is created if it does not exist yet
-
truncate – during opening of the file it is made empty (all content of the file is erased)
-
position at start – after file is opened, initial position is set to the start of the file
-
position at end – after file is opened, initial position is set to the end of the file
Note: a
and a+
always append to the end of file – ignores any seek
movements.
BTW. interesting behavior at least on my win7 / python2.7, for new file opened in a+
mode:
write('aa'); seek(0, 0); read(1); write('b')
– second write
is ignored
write('aa'); seek(0, 0); read(2); write('b')
– second write
raises IOError
I think this is important to consider for cross-platform execution, i.e. as a CYA. 🙂
On Windows, ‘b’ appended to the mode opens the file in binary mode, so there are also modes like ‘rb’, ‘wb’, and ‘r+b’. Python on Windows makes a distinction between text and binary files; the end-of-line characters in text files are automatically altered slightly when data is read or written. This behind-the-scenes modification to file data is fine for ASCII text files, but it’ll corrupt binary data like that in JPEG or EXE files. Be very careful to use binary mode when reading and writing such files. On Unix, it doesn’t hurt to append a ‘b’ to the mode, so you can use it platform-independently for all binary files.
This is directly quoted from Python Software Foundation 2.7.x.
I find it important to note that python 3 defines the opening modes differently to the answers here that were correct for Python 2.
The Python 3 opening modes are:
'r' open for reading (default)
'w' open for writing, truncating the file first
'x' open for exclusive creation, failing if the file already exists
'a' open for writing, appending to the end of the file if it exists
----
'b' binary mode
't' text mode (default)
'+' open a disk file for updating (reading and writing)
'U' universal newlines mode (for backwards compatibility; should not be used in new code)
The modes r
, w
, x
, a
are combined with the mode modifiers b
or t
. +
is optionally added, U
should be avoided.
As I found out the hard way, it is a good idea to always specify t
when opening a file in text mode since r
is an alias for rt
in the standard open()
function but an alias for rb
in the open()
functions of all compression modules (when e.g. reading a *.bz2
file).
Thus the modes for opening a file should be:
rt
/ wt
/ xt
/ at
for reading / writing / creating / appending to a file in text mode and
rb
/ wb
/ xb
/ ab
for reading / writing / creating / appending to a file in binary mode.
Use +
as before.
r | r+ | x | x+ | w | w+ | a | a+ | |
---|---|---|---|---|---|---|---|---|
readable | x | x | x | x | x | |||
writeable | x | x | x | x | x | x | x | |
default position: start | x | x | x | x | x | x | ||
default position: end | x | x | ||||||
must exist | x | x | ||||||
mustn’t exist | x | x | ||||||
truncate (clear file) on load | x | x | ||||||
Always write to EOF | x | x |
Mode
t (default) | b | |
---|---|---|
str (io.TextIOBase ) |
x | |
bytes (io.BufferedIOBase ) |
x |
If no mode is selected; text mode (t
) is used. As such r
is the same as rt
.
The particular doubt that many people get is ‘What is the difference between r+ and w+ modes?
The r+ helps you read and write data onto an already existing file without truncating (Error if there is no such file).
The w+ mode on the other hand also allows reading and writing but it truncates the file (if no such file exists – a new file is created). If you are wondering how it is possible to read from a truncated file, the reading methods can be used to read the newly written file (or the empty file).
Cheers!