Creating thumbnails from video files with Python
Question:
I need to create thumbnails for a video file once I’ve uploaded to a webapp running python.
How would I go about this… I need a library that can basically either do this for me, or that can read the image frames out of video files (of several formats) automatically.
Answers:
Look into PythonMagick, a Python interface to ImageMagick. That should have what you need. (Disclaimer: I haven’t used the Python interface before, but I know ImageMagick is good mojo.)
You could use the Youtube API for storage and transcoding and grab the feed thumbnails for free. Honestly, that’s the easiest way to handle online video and I’m not just shilling a 3rd party service, I’m a very happy user of that API and the internal video paths I was able to delete thanks to it.
You can use ffvideo
from ffvideo import VideoStream
pil_image = VideoStream('0.flv').get_frame_at_sec(5).image()
pil_image.save('frame5sec.jpeg')
import cv2
vcap = cv2.VideoCapture(filename)
res, im_ar = vcap.read()
while im_ar.mean() < threshold and res:
res, im_ar = vcap.read()
im_ar = cv2.resize(im_ar, (thumb_width, thumb_height), 0, 0, cv2.INTER_LINEAR)
#to save we have two options
#1) save on a file
cv2.imwrite(save_on_filename, im_ar)
#2)save on a buffer for direct transmission
res, thumb_buf = cv2.imencode('.png', im_ar)
# '.jpeg' etc are permitted
#get the bytes content
bt = thumb_buf.tostring()
“threshold” is an integer. When you get a video frame it can be very black, white etc to get some good thumbnail you can specify the mean value of all the pixel in the frame.
I could not install ffvideo on OSX Sierra so i decided to work with ffmpeg.
OSX:
brew install ffmpeg
Linux:
apt-get install ffmpeg
Python 3 Code:
import subprocess
video_input_path = '/your/video.mp4'
img_output_path = '/your/image.jpg'
subprocess.call(['ffmpeg', '-i', video_input_path, '-ss', '00:00:00.000', '-vframes', '1', img_output_path])
You can use the Python script pyvideothumbnailer found on GitHub, which uses PyAV, MediaInfo and PIL/Pillow. It was written by me and is available under the BSD-3-clause license. It should do the complete job for you.
It has meaningful defaults. So you can just start creating your first preview thumbnails image of an individual video file by invoking:
pyvideothumbnailer [VIDEO FILE]
or to create thumbnails of all video files located in the current working directory:
pyvideothumbnailer
or to create thumbnails of all video files located in a directory:
pyvideothumbnailer [DIRECTORY CONTAINING VIDEOS]
or in case that you want to create previews of videos in subdirectories as well:
pyvideothumbnailer --recursive [DIRECTORY CONTAINING VIDEOS]
Its behavior can be controlled by command line options and a user-defined configuration file .pyvideothumbnailer.conf.
Here are two examples how it works creating preview thumbmnails of Big Buck Bunny. For further reference have a look at the GitHub wiki page.
Using defaults:
pyvideothumbnailer bbb_sunflower_1080p_60fps_normal.mp4
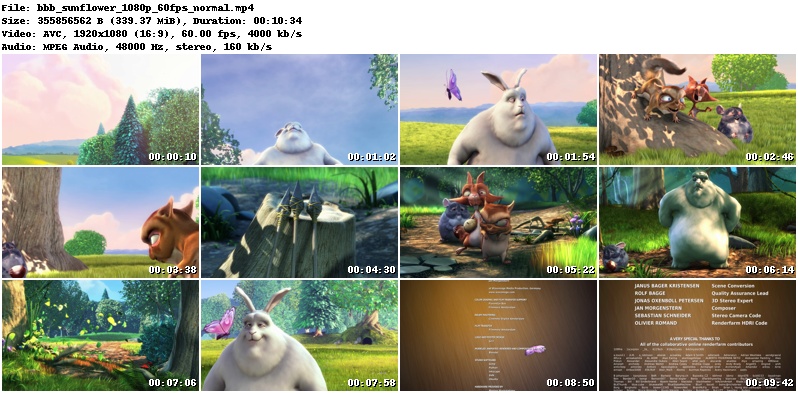
White header font on black background, DejaVuSans TrueType font instead of the built-in font, adding a comment at the bottom of the header, custom preview thumbnails image width and 5 x 4 preview thumbnails:
pyvideothumbnailer --background-color black --header-font-color white --header-font DejaVuSans.ttf --timestamp-font DejaVuSans.ttf --comment-text "Created with pyvideothumbnailer" --width 1024 --columns 5 --rows 4 bbb_sunflower_1080p_60fps_normal.mp4
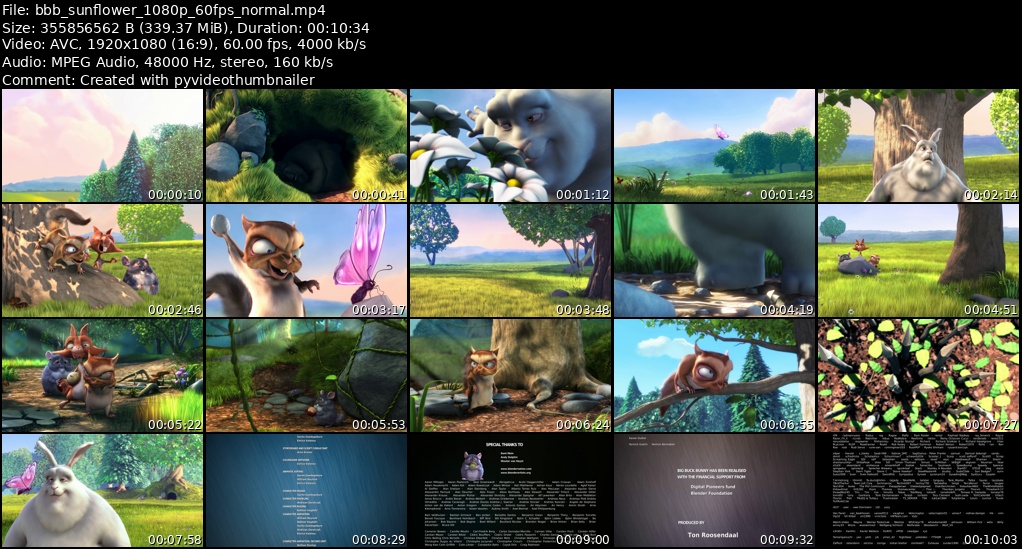
Use pyffmpeg
Install
pip install pyffmpeg
Use
from pyffmpeg import FFmpeg
inf = 'vid.mp4'
outf = 'thumb.jpg'
ff = FFmpeg()
ff.convert(inf, outf)
My Solution for both linux and windows os
-
windows download ffmeg compiled lib frmom https://www.gyan.dev/ffmpeg/builds/
add to environment variables
-
Linux apt-get install ffmpeg
-
Python Code
img_output_path = Path(img_output_path).absolute()
src_video_path = Path(src_video_path).absolute()
command = f"ffmpeg -i "{src_video_path}" -ss 00:00:00.000 -vframes 1 "{img_output_path}""
os.system(_command)
I need to create thumbnails for a video file once I’ve uploaded to a webapp running python.
How would I go about this… I need a library that can basically either do this for me, or that can read the image frames out of video files (of several formats) automatically.
Look into PythonMagick, a Python interface to ImageMagick. That should have what you need. (Disclaimer: I haven’t used the Python interface before, but I know ImageMagick is good mojo.)
You could use the Youtube API for storage and transcoding and grab the feed thumbnails for free. Honestly, that’s the easiest way to handle online video and I’m not just shilling a 3rd party service, I’m a very happy user of that API and the internal video paths I was able to delete thanks to it.
You can use ffvideo
from ffvideo import VideoStream
pil_image = VideoStream('0.flv').get_frame_at_sec(5).image()
pil_image.save('frame5sec.jpeg')
import cv2
vcap = cv2.VideoCapture(filename)
res, im_ar = vcap.read()
while im_ar.mean() < threshold and res:
res, im_ar = vcap.read()
im_ar = cv2.resize(im_ar, (thumb_width, thumb_height), 0, 0, cv2.INTER_LINEAR)
#to save we have two options
#1) save on a file
cv2.imwrite(save_on_filename, im_ar)
#2)save on a buffer for direct transmission
res, thumb_buf = cv2.imencode('.png', im_ar)
# '.jpeg' etc are permitted
#get the bytes content
bt = thumb_buf.tostring()
“threshold” is an integer. When you get a video frame it can be very black, white etc to get some good thumbnail you can specify the mean value of all the pixel in the frame.
I could not install ffvideo on OSX Sierra so i decided to work with ffmpeg.
OSX:
brew install ffmpeg
Linux:
apt-get install ffmpeg
Python 3 Code:
import subprocess
video_input_path = '/your/video.mp4'
img_output_path = '/your/image.jpg'
subprocess.call(['ffmpeg', '-i', video_input_path, '-ss', '00:00:00.000', '-vframes', '1', img_output_path])
You can use the Python script pyvideothumbnailer found on GitHub, which uses PyAV, MediaInfo and PIL/Pillow. It was written by me and is available under the BSD-3-clause license. It should do the complete job for you.
It has meaningful defaults. So you can just start creating your first preview thumbnails image of an individual video file by invoking:
pyvideothumbnailer [VIDEO FILE]
or to create thumbnails of all video files located in the current working directory:
pyvideothumbnailer
or to create thumbnails of all video files located in a directory:
pyvideothumbnailer [DIRECTORY CONTAINING VIDEOS]
or in case that you want to create previews of videos in subdirectories as well:
pyvideothumbnailer --recursive [DIRECTORY CONTAINING VIDEOS]
Its behavior can be controlled by command line options and a user-defined configuration file .pyvideothumbnailer.conf.
Here are two examples how it works creating preview thumbmnails of Big Buck Bunny. For further reference have a look at the GitHub wiki page.
Using defaults:
pyvideothumbnailer bbb_sunflower_1080p_60fps_normal.mp4
White header font on black background, DejaVuSans TrueType font instead of the built-in font, adding a comment at the bottom of the header, custom preview thumbnails image width and 5 x 4 preview thumbnails:
pyvideothumbnailer --background-color black --header-font-color white --header-font DejaVuSans.ttf --timestamp-font DejaVuSans.ttf --comment-text "Created with pyvideothumbnailer" --width 1024 --columns 5 --rows 4 bbb_sunflower_1080p_60fps_normal.mp4
Use pyffmpeg
Install
pip install pyffmpeg
Use
from pyffmpeg import FFmpeg
inf = 'vid.mp4'
outf = 'thumb.jpg'
ff = FFmpeg()
ff.convert(inf, outf)
My Solution for both linux and windows os
-
windows download ffmeg compiled lib frmom https://www.gyan.dev/ffmpeg/builds/
add to environment variables -
Linux
apt-get install ffmpeg
-
Python Code
img_output_path = Path(img_output_path).absolute() src_video_path = Path(src_video_path).absolute() command = f"ffmpeg -i "{src_video_path}" -ss 00:00:00.000 -vframes 1 "{img_output_path}"" os.system(_command)