React not showing POST response from FastAPI backend application
Question:
I have a simple React Ui which is supposed to get a json file from localhost:8000/todo
and create the Ui in localhost:3000
.
This is the desired output:
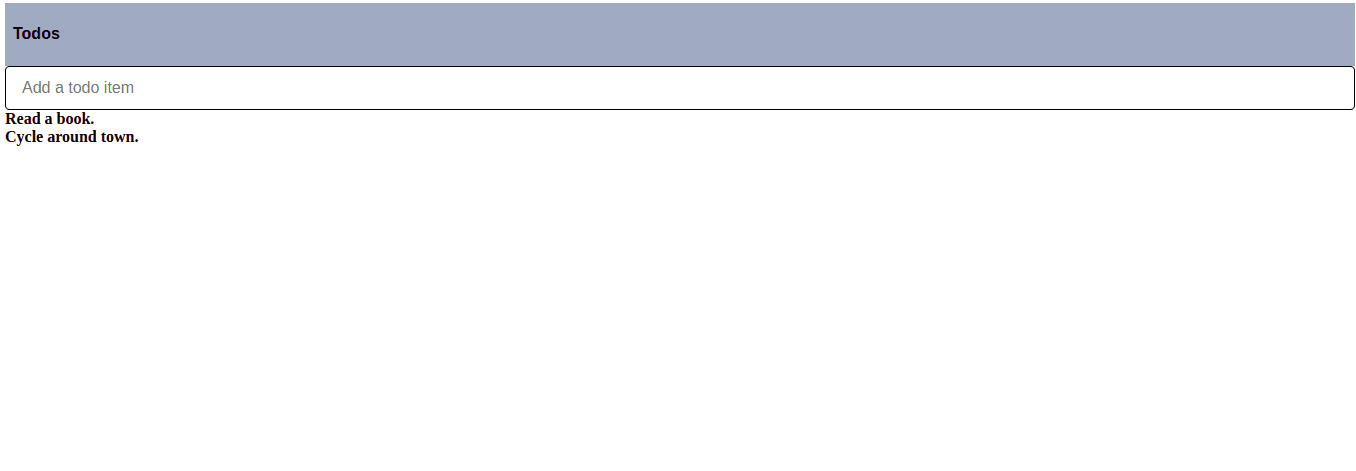
So, the two lines which are "Read a book." and "Cycle around town." are not shown. These two lines are supposed to come from localhost:8000/todo
which is a JSON
type information. I feel like I can fetch the data from localhost:8000/todo
, but I don’t know how to show them in localhost:3000
, which is my output.
Here is the function that I have for this:
export default function Todos() {
const [todos, setTodos] = useState([])
const fetchTodos = async () => {
const response = await fetch("http://localhost:8000/todo")
const todos = await response.json()
setTodos(todos.data)
}
useEffect(() => {
fetchTodos()
}, [])
return (
<TodosContext.Provider value={{todos, fetchTodos}}>
<AddTodo />
<Stack spacing={5}>
{todos.map((todo) => (
<b>{todo.item}</b>
))}
</Stack>
</TodosContext.Provider>
)
}
{todos.item} is the part that is supposed to print the items, but it doesn’t!
Here is the response from localhost:8000/todo
:

Please let me know if you need more information.
Answers:
You would need to enable CORS (Cross-Origin Resource Sharing) in the FastAPI backend. You can configure it in your FastAPI application using the CORSMiddleware
.
Note
Origin
An origin is the combination of protocol (http
, https
), domain
(myapp.com
, localhost
, localhost.tiangolo.com
), and port (80
,
443
, 8080
).
So, all these are different origins:
http://localhost
https://localhost
http://localhost:8080
Even if they are all in localhost
, they use different protocols or
ports, so, they are different "origins".
Example
Have a look at this related answer as well.
from fastapi import FastAPI
from fastapi.middleware.cors import CORSMiddleware
app = FastAPI()
origins = ["http://localhost:3000", "http://127.0.0.1:3000"]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)
I have a simple React Ui which is supposed to get a json file from localhost:8000/todo
and create the Ui in localhost:3000
.
This is the desired output:
So, the two lines which are "Read a book." and "Cycle around town." are not shown. These two lines are supposed to come from localhost:8000/todo
which is a JSON
type information. I feel like I can fetch the data from localhost:8000/todo
, but I don’t know how to show them in localhost:3000
, which is my output.
Here is the function that I have for this:
export default function Todos() {
const [todos, setTodos] = useState([])
const fetchTodos = async () => {
const response = await fetch("http://localhost:8000/todo")
const todos = await response.json()
setTodos(todos.data)
}
useEffect(() => {
fetchTodos()
}, [])
return (
<TodosContext.Provider value={{todos, fetchTodos}}>
<AddTodo />
<Stack spacing={5}>
{todos.map((todo) => (
<b>{todo.item}</b>
))}
</Stack>
</TodosContext.Provider>
)
}
{todos.item} is the part that is supposed to print the items, but it doesn’t!
Here is the response from localhost:8000/todo
:
Please let me know if you need more information.
You would need to enable CORS (Cross-Origin Resource Sharing) in the FastAPI backend. You can configure it in your FastAPI application using the CORSMiddleware
.
Note
Origin
An origin is the combination of protocol (
http
,https
), domain
(myapp.com
,localhost
,localhost.tiangolo.com
), and port (80
,
443
,8080
).So, all these are different origins:
http://localhost
https://localhost
http://localhost:8080
Even if they are all in
localhost
, they use different protocols or
ports, so, they are different "origins".
Example
Have a look at this related answer as well.
from fastapi import FastAPI
from fastapi.middleware.cors import CORSMiddleware
app = FastAPI()
origins = ["http://localhost:3000", "http://127.0.0.1:3000"]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)