How to add image to Swagger UI autodocs using FastAPI?
Question:
I would like to add an image to the FastAPI automatic documentation (provided by Swagger UI), but I can’t figure out how to do this.
This is the code:
@api.get(path='/carbon-credit/',
responses={
200: {'description': 'Ok',
"content": {
"image/jpeg": {
"example": 'https://picsum.photos/seed/picsum/200/300'
}
}},
404: {"description": "not found"},
422: {'description': 'not found 2'},
},
name='API for Carbon Credit',
description="get carbon credit",
tags=['Images'],
response_class=Response)
As you can see from the code, I’m trying to do this using a URL, and what I get in both ReDoc and Swagger UI is just the URL as a text, not the actual image. Also, I would like to use an image stored in the local drive.
Screenshots from Swagger UI and ReDoc:

How can I achieve that?
Thanks in advance.
Answers:
Both Swagger UI and ReDoc use standard HTML tags for the description
parameter. Hence, you can add the image using the description
.
@app.get(path='/',
responses={
200: {'description': '<img src="https://placebear.com/cache/395-205.jpg" alt="bear">',
...
OpenAPI also supports markdown elements (see here) and will render the image for you in Swagger UI/ReDoc. Hence, you can alternatively use:
@app.get(path='/',
responses={
200: {'description': '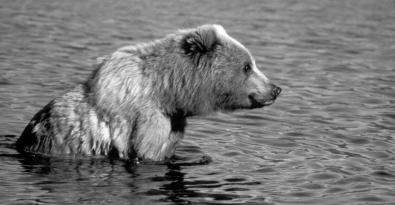',
...
To use an image stored in the local drive, you can mount a StaticFiles
instance to a specific path (let’s say /static
) to serve static files/images from a directory in your drive. Example below:
from fastapi import FastAPI
from fastapi.staticfiles import StaticFiles
app = FastAPI()
app.mount("/static", StaticFiles(directory="static"), name="static")
@app.get(path='/',
responses={
200: {'description': '',
...
Add the image to the examples field
To add the image to the examples
field you can again use the description
parameter, as shown below. Make sure you do that through the examples
parameter, not example
.
@app.get(path='/',
responses={
200: {"description": "OK",
"content": {
"image/jpeg": {
"schema": {
"type": "string",
"format": "binary"
},
"examples": {
"sampleImage": {
"summary": "A sample image",
"description": "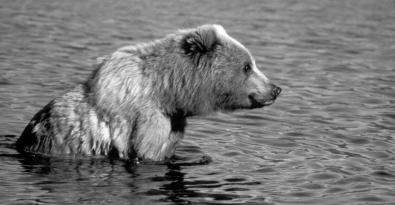",
"value": ""
}
}
}
}
},
404: {"description": "not found"},
422: {'description': 'not found 2'},
},
...
I would like to add an image to the FastAPI automatic documentation (provided by Swagger UI), but I can’t figure out how to do this.
This is the code:
@api.get(path='/carbon-credit/',
responses={
200: {'description': 'Ok',
"content": {
"image/jpeg": {
"example": 'https://picsum.photos/seed/picsum/200/300'
}
}},
404: {"description": "not found"},
422: {'description': 'not found 2'},
},
name='API for Carbon Credit',
description="get carbon credit",
tags=['Images'],
response_class=Response)
As you can see from the code, I’m trying to do this using a URL, and what I get in both ReDoc and Swagger UI is just the URL as a text, not the actual image. Also, I would like to use an image stored in the local drive.
Screenshots from Swagger UI and ReDoc:
How can I achieve that?
Thanks in advance.
Both Swagger UI and ReDoc use standard HTML tags for the description
parameter. Hence, you can add the image using the description
.
@app.get(path='/',
responses={
200: {'description': '<img src="https://placebear.com/cache/395-205.jpg" alt="bear">',
...
OpenAPI also supports markdown elements (see here) and will render the image for you in Swagger UI/ReDoc. Hence, you can alternatively use:
@app.get(path='/',
responses={
200: {'description': '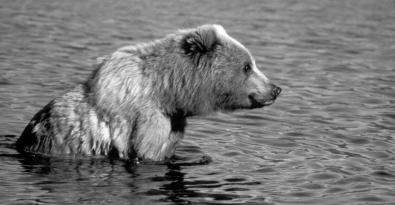',
...
To use an image stored in the local drive, you can mount a StaticFiles
instance to a specific path (let’s say /static
) to serve static files/images from a directory in your drive. Example below:
from fastapi import FastAPI
from fastapi.staticfiles import StaticFiles
app = FastAPI()
app.mount("/static", StaticFiles(directory="static"), name="static")
@app.get(path='/',
responses={
200: {'description': '',
...
Add the image to the examples field
To add the image to the examples
field you can again use the description
parameter, as shown below. Make sure you do that through the examples
parameter, not example
.
@app.get(path='/',
responses={
200: {"description": "OK",
"content": {
"image/jpeg": {
"schema": {
"type": "string",
"format": "binary"
},
"examples": {
"sampleImage": {
"summary": "A sample image",
"description": "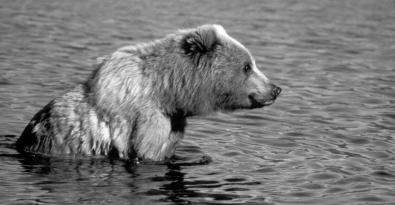",
"value": ""
}
}
}
}
},
404: {"description": "not found"},
422: {'description': 'not found 2'},
},
...